Amazing Python Package Showcase (1) – virtualenv
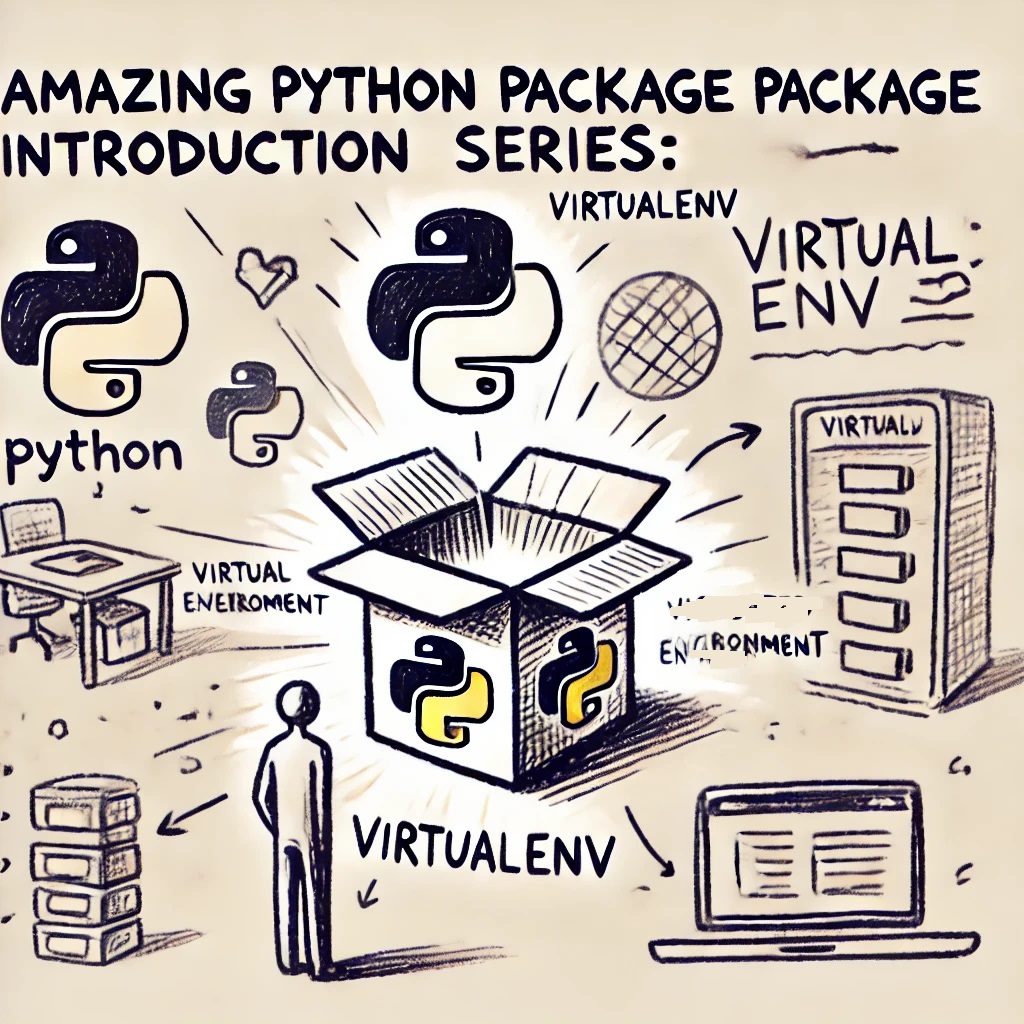
Table of Contents
Virtualenv is a powerful Python tool that enables the creation of isolated Python environments. This isolation ensures that dependencies from different projects do not interfere with each other, making it an essential tool for Python developers.
What is virtualenv?
Virtualenv is a tool to create isolated Python environments. It helps manage project-specific dependencies without affecting the system Python installation or other projects. You can find it at https://virtualenv.pypa.io/en/latest/index.html
Installing virtualenv
To install virtualenv, use pip:
$ pip install virtualenv
Defaulting to user installation because normal site-packages is not writeable
Collecting virtualenv
Downloading virtualenv-20.26.3-py3-none-any.whl (5.7 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 5.7/5.7 MB 3.6 MB/s eta 0:00:00
Collecting distlib<1,>=0.3.7
Downloading distlib-0.3.8-py2.py3-none-any.whl (468 kB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 468.9/468.9 KB 3.6 MB/s eta 0:00:00
Collecting filelock<4,>=3.12.2
Downloading filelock-3.15.4-py3-none-any.whl (16 kB)
Collecting platformdirs<5,>=3.9.1
Downloading platformdirs-4.2.2-py3-none-any.whl (18 kB)
Installing collected packages: distlib, platformdirs, filelock, virtualenv
Successfully installed distlib-0.3.8 filelock-3.15.4 platformdirs-4.2.2 virtualenv-20.26.3
Creating a Virtual Environment
To create a virtual environment, navigate to your project directory and run:
$ virtualenv myenv
created virtual environment CPython3.10.12.final.0-64 in 927ms
creator CPython3Posix(dest=/home/dynotes/myenv, clear=False, no_vcs_ignore=False, global=False)
seeder FromAppData(download=False, pip=bundle, setuptools=bundle, wheel=bundle, via=copy, app_data_dir=/home/dynotes/.local/share/virtualenv)
added seed packages: pip==24.1, setuptools==70.1.0, wheel==0.43.0
activators BashActivator,CShellActivator,FishActivator,NushellActivator,PowerShellActivator,PythonActivator
This command creates a directory named myenv
containing a standalone Python installation.
Activating and Deactivating the Virtual Environment
Activate the environment:
On Windows
myenv\Scripts\activate
On macOS and Linux
source myenv/bin/activate
Deactivate the environment
dynotes@P2021:~$ source myenv/bin/activate
(myenv) dynotes@WIN-P2021:~$ deactivate
dynotes@P2021:~$
Installing Packages in the Virtual Environment
Once the environment is activated, you can install packages using pip install package_name
(myenv) dynotes@P2021:~/projects/python/numpy$ pip install numpy
Collecting numpy
Downloading numpy-2.0.0-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl.metadata (60 kB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 60.9/60.9 kB 1.4 MB/s eta 0:00:00
Downloading numpy-2.0.0-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (19.3 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 19.3/19.3 MB 3.6 MB/s eta 0:00:00
Installing collected packages: numpy
Successfully installed numpy-2.0.0
The packages installed will only be available in the virtual environment.
Example Script
Here’s an example script that you can use within your virtual environment
import numpy as np
a = np.arange(15).reshape(3, 5)
print(a)
print(a.shape)
print(a.ndim)
print(a.dtype.name)
print(a.itemsize)
print(a.size)
To run the script, in the activated environment execute
(myenv) dynotes@P2021:~/projects/python/numpy$ python example1.py
[[ 0 1 2 3 4]
[ 5 6 7 8 9]
[10 11 12 13 14]]
(3, 5)
2
int64
8
15
Different Tools for Creating Isolated Python Environments
virtualenv vs Conda
Virtualenv
- Purpose: Primarily for creating isolated Python environments.
- Package Management: Uses
pip
to install Python packages. - Language Support: Only Python.
- Environment Management: Simple, lightweight, and quick to set up.
- Dependency Management: Relies on
requirements.txt
for managing dependencies. - Activation: Requires manual activation using commands like
source venv/bin/activate
(Linux/Mac) orvenv\Scripts\activate
(Windows).
Conda
- Purpose: Manages environments and packages for multiple languages (Python, R, Ruby, etc.).
- Package Management: Uses
conda
to install packages, which can include non-Python dependencies. - Language Support: Multi-language support.
- Environment Management: More comprehensive, can handle complex dependencies.
- Dependency Management: Uses
environment.yml
to manage dependencies, which can include non-Python packages. - Activation: Uses
conda activate <env_name>
for environment activation.
Key Differences
- Scope: Virtualenv is focused on Python, while Conda supports multiple languages and broader environment management.
- Package Sources: Virtualenv relies on PyPI, whereas Conda has its own package repositories that include a wide range of software, including system libraries and tools.
- Complexity: Conda is more complex but offers greater flexibility, especially for data science and scientific computing where multiple types of dependencies are common.
virtualenv and python -m venv
virtualenv
- Purpose: A third-party tool for creating isolated Python environments.
- Compatibility: Works with Python 2 and 3.
- Features: More features and flexibility (e.g., can create environments with different versions of Python).
- Installation: Requires installation via pip (
pip install virtualenv
).
python -m venv
- Purpose: A built-in module in Python 3.3 and later for creating isolated environments.
- Compatibility: Only works with Python 3.3+.
- Features: Simplified and part of the Python standard library.
Key Differences
virtualenv
offering more flexibility andpython -m venv
being more straightforward and part of the standard library for Python 3.3+.
Note: all command lines are run in WLS 2,. If you are interested in WLS 2, please refer to https://templespark.com/master-wsl-2-a-concise-guide-for-windows-subsystem-for-linux/
Leave a Reply