Amazing Python Package Showcase (5) – Mimesis: Fake Data Generator
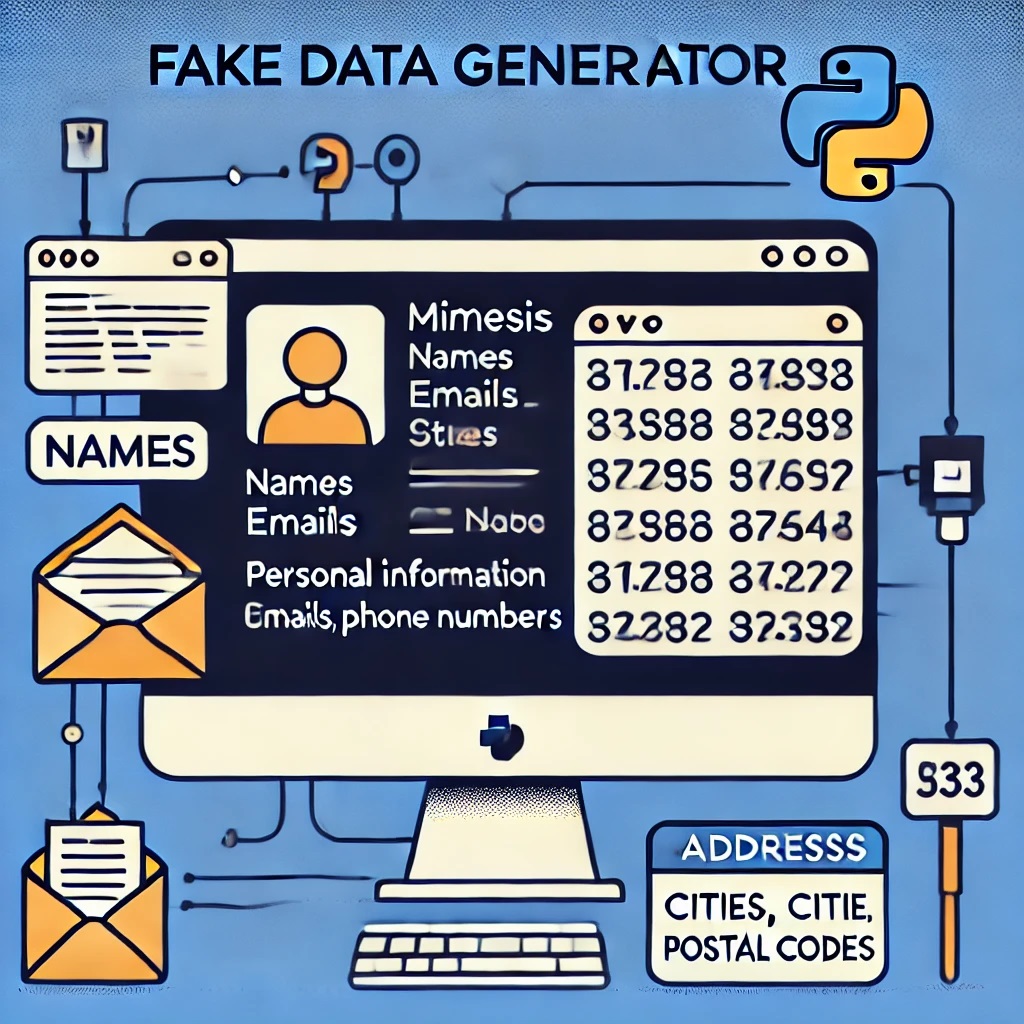
In this fifth article of the “Amazing Python Package Showcase Series,” we explore Mimesis, a powerful library designed to generate fake data for various purposes. Whether you’re testing applications, filling databases, or performing data analysis, Mimesis provides a vast array of options to create realistic fake data efficiently.
Table of Contents
What is Mimesis?
Mimesis is a Python library that simplifies the process of generating fake data. It’s highly customizable and supports various data types and locales, making it an ideal choice for developers and data scientists who need to create large datasets quickly and easily.
Key Features
- Wide Range of Data Types: Mimesis can generate names, addresses, dates, numbers, text, and more.
- Localization Support: Supports multiple locales, allowing you to generate data in different languages and formats.
- Custom Providers: Extend Mimesis with custom data providers to fit specific needs.
- Integration Ready: Easy to integrate with other Python libraries and frameworks.
Installation
To get started with Mimesis, create an environment first:
$ virtualenv mimesis
created virtual environment CPython3.10.12.final.0-64 in 550ms
creator CPython3Posix(dest=/home/dynotes/projects/python/mimesis/mimesis, clear=False, no_vcs_ignore=False, global=False)
seeder FromAppData(download=False, pip=bundle, setuptools=bundle, wheel=bundle, via=copy, app_data_dir=/home/dynotes/.local/share/virtualenv)
added seed packages: pip==24.1.2, setuptools==70.1.1, wheel==0.43.0
activators BashActivator,CShellActivator,FishActivator,NushellActivator,PowerShellActivator,PythonActivator
$ source ./mimesis/bin/activate
(mimesis) dynotes@P2021:~/projects/python/mimesis$
Then install mimesis
via pip
:
(mimesis) dynotes@P2021:~/projects/python/mimesis$ pip install mimesis
Collecting mimesis
Downloading mimesis-17.0.0-py3-none-any.whl.metadata (5.7 kB)
Downloading mimesis-17.0.0-py3-none-any.whl (4.5 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 4.5/4.5 MB 9.4 MB/s eta 0:00:00
Installing collected packages: mimesis
Successfully installed mimesis-17.0.0
Examples
Basic Usage
Here’s a simple example to demonstrate how to generate fake data using Mimesis:
from mimesis import Person
from mimesis.enums import Gender
from mimesis.locales import Locale
# Create a person object with a specific locale
person = Person(Locale.EN)
# Generate fake data
print(f"Name: {person.full_name(gender=Gender.MALE)}")
print(f"Email: {person.email()}")
print(f"Phone: {person.telephone()}")
Save the above code into a file named as example1.py
and run it:
(mimesis) dynotes@P2021:~/projects/python/mimesis$ python example1.py
Name: Jaime Strong
Email: fork2015@example.com
Phone: +1-573-299-9192
Advanced Usage – A Custom Provider Example
Mimesis offers advanced features to customize and extend its functionality. You can create custom providers to generate specific types of data not covered by the built-in providers.
from mimesis import Generic
from mimesis.providers import BaseProvider
class MyProvider(BaseProvider):
def custom_field(self):
return 'Custom Data Provided by Mimesis'
generic = Generic()
generic.add_provider(MyProvider)
print(generic.myprovider.custom_field())
Save the above code into a file named as example2.py
and run it:
(mimesis) dynotes@P2021:~/projects/python/mimesis$ python example2.py
Custom Data Provided by Mimesis
Full Practical Example: Generating User Profiles
In this example, we will use Mimesis to generate fake user profiles, including names, emails, addresses, and phone numbers. We will then save this data to a CSV file, which can be used for testing purposes in a user management application.
import csv
from mimesis import Person, Address, Datetime
from mimesis.enums import Gender
from mimesis.locales import Locale
# Function to generate a list of user profiles
def generate_user_profiles(num_profiles):
person = Person(Locale.EN)
address = Address(Locale.EN)
datetime = Datetime(Locale.EN)
user_profiles = []
for _ in range(num_profiles):
profile = {
"Name": person.full_name(gender=Gender.MALE if _ % 2 == 0 else Gender.FEMALE),
"Email": person.email(),
"Phone": person.telephone(),
"Address": f"{address.street_name()} {address.street_number()}, {address.city()}, {address.postal_code()}",
"Birthdate": datetime.date(start=1970, end=2000)
}
user_profiles.append(profile)
return user_profiles
# Function to save user profiles to a CSV file
def save_profiles_to_csv(profiles, filename):
fieldnames = ["Name", "Email", "Phone", "Address", "Birthdate"]
with open(filename, mode='w', newline='') as file:
writer = csv.DictWriter(file, fieldnames=fieldnames)
writer.writeheader()
for profile in profiles:
writer.writerow(profile)
# Generate 100 user profiles
num_profiles = 100
profiles = generate_user_profiles(num_profiles)
# Save the profiles to a CSV file
csv_filename = "user_profiles.csv"
save_profiles_to_csv(profiles, csv_filename)
print(f"Generated {num_profiles} user profiles and saved to {csv_filename}")
Save the above code into a file named as practical_usecase.py
and run it:
(mimesis) dynotes@P2021:~/projects/python/mimesis$ python practical_usecase.py
Generated 100 user profiles and saved to user_profiles.csv
Here are the results saved on the file of user_profiles.csv
:
Name,Email,Phone,Address,Birthdate
Bennie Mcmahon,kernel1838@yahoo.com,+12275254319,"Hardie 1236, Los Angeles, 29134",1998-10-24
Annalisa Howell,plans2038@live.com,+1-626-572-3071,"Granada 28, Missoula, 39944",1980-10-17
Carmelo Scott,toolbar1919@example.com,+12408004140,"Majestic 1253, Tamarac, 53709",1993-01-18
Wan Boyer,awarded1882@gmail.com,+1-606-526-1412,"Maywood 922, Waterbury, 83187",1979-12-18
Roberto Strickland,factory1822@example.org,+1-662-521-9645,"San Pablo 38, Plantation, 22565",1982-04-21
Breann Gonzales,using2086@outlook.com,+1-323-462-6349,"Riverton 506, Spring Valley, 21966",1994-07-27
Walter Cunningham,playstation1900@example.org,+14067194571,"Almaden 301, Derby, 94806",1970-09-24
Hae Hensley,humanity1964@yahoo.com,+1-814-210-1527,"Edward 852, Eagle, 35949",1981-06-16
Otto Calhoun,opposed1903@yandex.com,+12282626870,"Ramona 290, San Carlos, 46350",1992-03-16
Norah Bernard,sexually1975@live.com,+12098254783,"Flora 956, Palm Desert, 42400",1997-09-09
Forest Franks,looksmart1972@live.com,+1-985-544-1413,"Hawkins 745, Hampton, 13617",1972-11-23
Giuseppina Knapp,cookie1856@live.com,+15136326713,"Jordan 201, Aiken, 00253",1979-03-28
Dannie Mcguire,guys1885@protonmail.com,+1-712-054-0812,"Garlington 1116, New Rochelle, 75162",1974-08-17
Sheilah Barron,eclipse2028@duck.com,+1-571-880-7072,"Storrie 129, La Cañada Flintridge, 60753",1998-11-25
Aldo Patton,promotion2083@example.org,+12033234777,"Jefferson 229, Elko, 75386",1978-09-19
Karima Simon,spanish2023@yahoo.com,+12241442412,"Abbey 60, Stanton, 89332",1981-10-30
Sang Rasmussen,anthony1899@yahoo.com,+1-430-726-0750,"Van Dyke 1081, Edmond, 97634",1980-07-13
Marcelene Branch,methodology2051@live.com,+12243561257,"Brosnan 955, Safety Harbor, 99365",1988-07-21
Trey West,cookies1829@duck.com,+17321116808,"Jersey 741, Tempe, 00734",1992-09-08
Conchita Harper,committees1847@duck.com,+18357232585,"Halibut 191, Lynbrook, 55733",1994-05-22
Rhett Gay,alleged2089@example.com,+1-865-861-8519,"Evans 59, Harrisonburg, 21148",1989-02-05
Marybelle Burks,its2025@duck.com,+17344862382,"Elk 1389, Baton Rouge, 41587",2000-11-22
Nathaniel Arnold,provides1995@duck.com,+12140619503,"Alton 1308, Muncie, 09839",1983-01-24
Janee Steele,task2098@example.org,+1-906-557-0565,"Dwight 485, Federal Way, 38858",1983-06-20
Maxwell Harrell,suse1939@example.org,+1-231-187-0391,"Sansome 42, Kettering, 93168",1994-01-23
Shan Clark,cornwall1906@example.org,+14094523074,"Seminole 676, Manhattan Beach, 73136",1979-02-20
Grady English,canvas1887@live.com,+1-201-045-0602,"Avenue M 1165, Thomasville, 40226",1992-05-24
Carolyne Lucas,divided2048@yandex.com,+18100812872,"Evelyn 1285, Ferguson, 41414",1976-07-10
Raphael Marquez,thank1855@example.org,+15208571426,"San Marcos 1070, Missouri City, 19875",1975-05-14
Quyen Mcgee,inquiry2086@yahoo.com,+17153474107,"Petrarch 326, Alamo, 76978",1971-06-05
Freddy Gould,bidder1805@outlook.com,+17042576401,"Ludlow 1085, Corvallis, 29153",1991-01-20
Marilu Hansen,ends2091@example.com,+13399907520,"Nautilus 1037, Pharr, 15806",1974-11-14
Efren Williamson,tiger1818@example.org,+16078628463,"Morgan 1337, Warner Robins, 64772",1993-02-15
Laquanda Bright,finite2010@duck.com,+1-316-744-2216,"Gerard 45, Burbank, 40646",1982-08-17
Alden Guerra,wrong1825@yandex.com,+1-701-962-9623,"Alviso 1277, North Miami Beach, 75414",1970-01-19
Danae Emerson,msgstr1879@live.com,+1-254-775-0819,"Ewer 1133, Sugar Land, 88356",1973-07-06
Lawrence Patterson,legend1969@protonmail.com,+19560562708,"Fresnel 59, Covington, 55852",1988-03-17
Nadene Logan,cart2049@yandex.com,+19846184735,"Ophir 1161, Fond du Lac, 47452",1994-11-05
Buck Kramer,duck2001@duck.com,+1-843-878-0263,"Harvard 121, Mesa, 35348",2000-09-24
Kathaleen Hayes,safely1904@example.org,+19177619924,"Ford 413, Fresno, 08826",1998-11-10
Casey Shaffer,industrial2065@example.org,+18356495464,"Funston 1358, Angleton, 89316",1990-01-15
Olympia Potter,necklace1933@example.org,+1-734-670-9547,"Wilde 12, Lee's Summit, 54125",1973-01-01
Todd Nelson,bacon2011@example.com,+13095411001,"Antonio 463, Rancho Mirage, 30362",1985-02-19
Tyler Tate,birmingham2063@example.com,+1-906-466-7803,"Spruce 1329, Fairmont, 91103",1984-07-28
Mario Jefferson,modified2037@outlook.com,+1-927-453-6783,"Agua 808, Oak Forest, 51341",1981-04-02
Emmaline Best,beautiful1968@gmail.com,+12135698194,"Nueva 683, Wilkinsburg, 12742",1989-06-21
Pablo Donovan,enemy1937@example.org,+1-308-647-3253,"August 102, Niles, 23289",1970-11-12
Delisa Hyde,kidney2051@example.com,+1-369-033-7302,"Stoneman 874, DeSoto, 33637",1991-06-29
Foster Clark,anti1831@protonmail.com,+17132983188,"Arkansas 695, Steubenville, 09170",1976-01-30
Cythia Soto,threads2012@gmail.com,+1-540-863-2689,"Merced 422, Nogales, 70342",1982-02-01
Giuseppe Parks,integrating1913@outlook.com,+1-213-242-3633,"Vicksburg 468, Hammond, 33877",1980-01-31
Britni Silva,desperate2015@yandex.com,+19081504268,"Patterson 1111, Issaquah, 87862",2000-05-14
Eddie Robles,rim1977@gmail.com,+1-623-407-9852,"Davidson 1074, Hialeah, 23037",1982-08-16
Bernarda Strong,dairy2096@yahoo.com,+14192386017,"Hidalgo 973, Wadsworth, 20815",1991-04-30
Elliot Jones,talks2034@yandex.com,+1-773-366-4884,"Dukes 63, Phoenix, 85275",1974-10-13
Breana Hayden,ensemble1998@outlook.com,+1-507-878-9601,"Onique 848, Apopka, 34906",1990-03-08
Josh Bailey,matters1849@yandex.com,+15158615658,"Lowell 671, State College, 40412",1988-03-13
Tambra Orr,seat1913@yahoo.com,+1-530-473-9960,"Moulton 1282, Pine Bluff, 10833",1974-10-05
Matt Bentley,painful2011@example.com,+18152979462,"Holladay 1381, South Burlington, 42967",1974-11-11
Deane Alexander,facilitate1922@gmail.com,+15046442894,"Henry Adams 1096, Flint, 86135",1975-02-11
Alfredo Hayden,choir2007@protonmail.com,+19200442854,"Garces 937, Poughkeepsie, 97805",1992-01-20
Floretta Nichols,cloud1908@outlook.com,+14300111002,"Fort Mason 363, Millville, 19578",1977-01-21
Sandy Thornton,legacy2019@yandex.com,+1-704-540-4945,"Foote 930, Ashwaubenon, 37080",1973-11-04
Merri Greene,marked2100@yahoo.com,+14345293233,"Sylvan 593, Emporia, 62933",1986-01-27
Chuck Elliott,ministers1971@live.com,+12839134412,"Bonita 987, Charlottesville, 48109",1986-09-02
Kaylene Herring,acquire1942@duck.com,+16797971467,"Infantry 1199, St. Charles, 62810",1997-05-14
Michal Walsh,piece1816@gmail.com,+19385467711,"Harlow 1360, Villa Park, 73081",1972-07-29
Jong Boyd,ntsc1989@example.com,+16071731851,"Isadora Duncan 1102, Eagle Mountain, 03114",1987-09-08
Joey Ballard,promise1832@protonmail.com,+16142267004,"Quickstep 568, Norwalk, 59017",1982-10-30
Catheryn Daniel,updated1859@duck.com,+15701060678,"Ankeny 998, Laurel, 78898",1974-01-06
Nestor Rosario,novelty1862@yahoo.com,+1-225-403-3288,"Villa 517, Mesa, 16687",1974-10-06
Roseline Levine,dated2026@example.com,+12563372349,"Turner 229, Geneva, 74740",1987-05-11
Alfred Ford,das1910@live.com,+18131032831,"Paul 1313, Tuscaloosa, 41223",1977-05-20
Tiny Clayton,yellow1927@duck.com,+18289497241,"Burritt 82, Eugene, 46790",1975-12-30
Harland Parsons,incentives2030@duck.com,+1-502-829-4896,"De Montfort 1208, Wausau, 40386",1999-10-04
Denese Collier,endorsed1932@yahoo.com,+1-916-094-7664,"Encinal 1196, Crown Point, 34968",1997-12-20
Jody Hale,palmer2093@protonmail.com,+1-360-600-9060,"Candlestick Cove 864, Cambridge, 93496",1981-05-17
Felisa Harris,coat2020@outlook.com,+1-863-819-9994,"Macalla 1238, Hawthorne, 83051",1987-11-23
Paul Buck,advice2036@protonmail.com,+1-405-885-0616,"Dunshee 515, Danbury, 12330",1989-07-02
Hedy Mills,bikes1884@duck.com,+17153689589,"Alta 116, Greenville, 42071",1990-04-11
Irwin Marquez,rack2054@gmail.com,+17736389040,"Seawell 525, Sunrise, 40289",1973-05-29
Heike Aguirre,outdoor1854@duck.com,+1-281-972-1182,"Giants 59, Waterbury, 08439",1975-04-11
Davis Hatfield,performed2057@gmail.com,+13010593778,"Maple 465, Delaware, 56975",1975-03-04
Setsuko Finley,revolution1965@outlook.com,+1-720-443-0725,"Larch 388, Somerville, 46555",1985-02-06
Neville Mcdonald,win1831@example.com,+1-424-320-0646,"Murray 1004, Los Altos, 66576",1998-03-01
Cherish Nicholson,obesity2077@example.org,+18353359251,"Essex 455, Santa Barbara, 08236",1987-05-01
Damian Schmidt,behavioral1988@duck.com,+1-831-705-6540,"Margrave 1071, Santa Monica, 98432",1985-01-16
Ehtel Noel,plc1822@example.com,+1-562-294-0568,"Waterloo 1329, South Lake Tahoe, 66419",1980-10-23
Stacy Mcguire,institute1832@yandex.com,+13192953374,"Shafter 1374, Kent, 34693",1987-04-18
Jon Thomas,reform2016@example.org,+1-470-502-4878,"Saint George 854, Newburyport, 11524",1971-05-29
Javier Witt,holes2028@example.org,+1-571-538-1749,"El Camino Del Mar 691, Pasco, 27106",1998-11-23
Emilee Garner,silicon1898@live.com,+1-775-925-2095,"Kendall 1000, Lewiston, 13167",1980-11-16
Mickey Donaldson,chart1961@protonmail.com,+19416528137,"Gorham 409, Perris, 14333",1974-04-02
Sunday England,origin2086@outlook.com,+17543527534,"Hilton 1193, Clearfield, 57939",1990-08-18
Cornell Maddox,completion1816@gmail.com,+19189996050,"Shoup 687, Sanford, 80591",2000-03-16
Ronald Byrd,robertson2046@duck.com,+12316626693,"Presidio 472, Bonney Lake, 67992",1980-03-09
Terry Patterson,embassy1989@gmail.com,+12742835976,"Dellbrook 18, Galveston, 88216",1976-12-13
Janae Mitchell,shaft1976@example.com,+15646717913,"Cortes 629, Mission, 79809",1990-10-04
Greg Bishop,pike1965@example.org,+1-207-424-8535,"Rockaway 981, Richland, 41220",1990-03-29
Carmon Johns,speeds2025@duck.com,+13232240818,"Worden 1304, Belleville, 59749",1994-08-07
List of Main Providers
Below is a summary of the primary providers provided by Mimesis, categorized by the type of data they generate:
Basic Data Providers
- Person: Generate personal information such as names, emails, phone numbers, etc.
- Address: Generate addresses, including streets, cities, postal codes, etc.
- Datetime: Generate dates and times.
- Text: Generate random text, sentences, paragraphs, etc.
- Numbers: Generate random numbers, integers, floats, etc.
Specialized Data Providers
- Business: Generate business-related data such as company names, job titles, etc.
- Science: Generate scientific data such as chemical elements, planets, etc.
- Food: Generate food-related data such as fruits, vegetables, dishes, etc.
- Internet: Generate internet-related data such as URLs, IP addresses, etc.
- Finance: Generate financial data such as currency codes, credit card numbers, etc.
- File: Generate file-related data such as file names, MIME types, etc.
- Hardware: Generate hardware-related data such as CPU models, GPU models, etc.
- Payment: Generate payment-related data such as credit card numbers, payment methods, etc.
- Development: Generate data related to software development such as programming languages, IDEs, etc.
- Unit System: Generate units of measurement such as lengths, weights, temperatures, etc.
Mimesis is a versatile and powerful tool for generating fake data in Python. Its extensive features and ease of use make it a valuable addition to any developer or data scientist’s toolkit. Whether you’re testing, analyzing, or developing, Mimesis can help you create the data you need quickly and efficiently.
Leave a Reply