Python F-Strings Explained: Efficient and Readable String Formatting – Amazing Python Package Showcase (8)
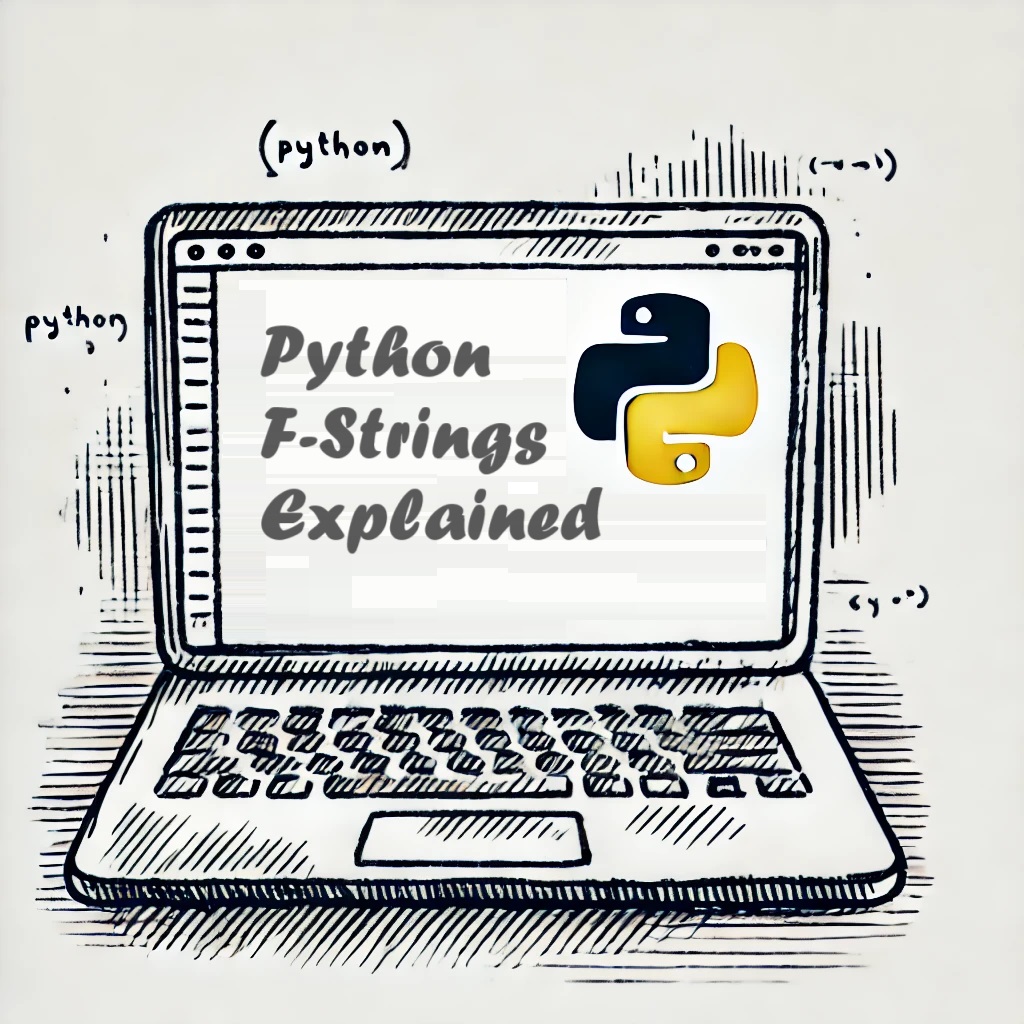
Table of Contents
Python, known for its simplicity and readability, introduced a powerful feature in version 3.6: formatted string literals, commonly known as f-strings. F-strings provide a concise and readable way to embed expressions inside string literals, making string formatting more intuitive and efficient.
What Are F-Strings?
F-strings are string literals prefixed with f
or F
, allowing expressions to be embedded within curly braces {}
. This feature enhances readability and reduces the complexity of string formatting.
Basic Usage
Creating an f-string is straightforward. Here’s a simple example:
name = "Alice"
age = 30
greeting = f"Hello, my name is {name} and I am {age} years old."
print(greeting)
This will output:
Hello, my name is Alice and I am 30 years old.
Expressions Inside F-Strings
F-strings can include any valid Python expression, making them highly versatile:
import math
result = f"The square root of 16 is {math.sqrt(16)}."
print(result)
This will output:
The square root of 16 is 4.0.
Formatting Numbers
F-strings support various formatting options, similar to the str.format()
method. For example, to format a floating-point number to two decimal places:
value = 123.456
formatted_value = f"{value:.2f}"
print(formatted_value) # Outputs: 123.46
To include a thousands separator:
value = 1234567.89
formatted_value = f"{value:,.2f}"
print(formatted_value) # Outputs: 1,234,567.89
Formatting Dates
You can also format dates using the datetime
module:
from datetime import datetime
now = datetime.now()
formatted_date = f"{now:%Y-%m-%d %H:%M:%S}"
print(formatted_date) # Outputs: '2024-08-21 19:05:48'
Escaping Curly Braces
To include literal curly braces in your f-string, double them:
escaped_string = f"Curly braces can be escaped like this: {{}}"
print(escaped_string) # Outputs: Curly braces can be escaped like this: {}
Practical Example: Generating a Sales Report
Scenario
Imagine you have a list of sales data for different products, and you want to generate a formatted report that includes the product name, units sold, and total revenue. Here’s how you can use f-strings to achieve this:
from datetime import datetime
# Sample sales data
sales_data = [
{"product": "Laptop", "units_sold": 50, "unit_price": 1200.00},
{"product": "Smartphone", "units_sold": 150, "unit_price": 800.00},
{"product": "Tablet", "units_sold": 100, "unit_price": 600.00},
]
# Generate the report
report_date = datetime.now()
report = f"Sales Report - {report_date:%Y-%m-%d}\n"
report += "=" * 40 + "\n"
report += f"{'Product':<15}{'Units Sold':<15}{'Total Revenue':<15}\n"
report += "-" * 40 + "\n"
for item in sales_data:
product = item["product"]
units_sold = item["units_sold"]
total_revenue = units_sold * item["unit_price"]
report += f"{product:<15}{units_sold:<15}{total_revenue:<15,.2f}\n"
report += "=" * 40 + "\n"
print(report)
Explanation
- Data Preparation: We have a list of dictionaries containing sales data for different products.
- Report Header: We use an f-string to include the current date in the report header.
- Table Header: We format the table headers (
Product
,Units Sold
,Total Revenue
) with left alignment and a fixed width. - Data Rows: For each item in the sales data, we calculate the total revenue and format the row using f-strings. The
:<15
ensures left alignment with a width of 15 characters, and,.2f
formats the total revenue with commas and two decimal places. - Output: The report is printed with a formatted structure.
Output
The output will look something like this:
Sales Report - 2024-08-21
========================================
Product Units Sold Total Revenue
----------------------------------------
Laptop 50 60,000.00
Smartphone 150 120,000.00
Tablet 100 60,000.00
========================================
More Considerations
Advantages of F-Strings
F-strings offer several advantages over other string formatting methods:
- Readability: F-strings are more readable and concise.
- Performance: They are generally faster than the
%
operator andstr.format()
. - Ease of Use: Direct inclusion of expressions simplifies the code.
- Debugging: F-strings support a special syntax for debugging.
- Multiline Support: They can be used with triple quotes for multiline strings.
Limitations of F-Strings
Despite their benefits, f-strings have some limitations:
Python 3.6+ Only
F-strings are only available in Python 3.6 and later versions. If you need to support older versions of Python, you’ll have to use other string formatting methods like %
or str.format()
.
No Support for Backslashes
F-strings do not support backslashes directly within the curly braces. This can be a limitation when you need to include escape sequences.
# This will raise a SyntaxError
path = f"C:\Users\{username}\Documents"
To work around this, you can use double backslashes or raw strings:
path = f"C:\\Users\\{username}\\Documents"
# or
path = fr"C:\Users\{username}\Documents"
Limited to Expressions
F-strings can only evaluate expressions, not statements. This means you can’t include assignments or other statements inside the curly braces.
# This will raise a SyntaxError
value = f"{x = 10}"
Complexity in Nested Braces
Using nested braces can be tricky and may lead to readability issues.
# This can be confusing
nested = f"{{ {value} }}"
No Deferred Evaluation
F-strings evaluate expressions at runtime, which means they don’t support deferred evaluation. If you need to delay the evaluation of an expression, you’ll need to use a different approach.
Security Concerns
If you’re using f-strings with user input, be cautious of security risks like code injection. Always validate and sanitize user input to avoid potential vulnerabilities.
Scenario
Consider a scenario where you use f-strings to log user input directly:
user_input = "some_input"
log_message = f"User input: {user_input}"
print(log_message)
If user_input
is controlled by an attacker, they could potentially inject malicious code. For example:
import os
log_message = f"User input: {os.system('rm -rf /')}" # DON'T RUN THIS LINE!!!
print(log_message)
In this case, the f-string will evaluate the expression inside the curly braces, potentially executing the injected code, which could be catastrophic.
Mitigating the Risk
To mitigate this risk, always sanitize and validate user input before using it in f-strings. Alternatively, use safer methods for handling untrusted data, such as template strings from the string
module, which do not evaluate expressions:
from string import Template
user_input = "some_input"
template = Template("User input: $user_input")
log_message = template.safe_substitute(user_input=user_input)
print(log_message)
This approach ensures that the user input is treated as plain text and not as executable code.
Summary
F-strings are a powerful addition to Python, making string formatting more intuitive and efficient. Whether you’re formatting numbers, dates, or embedding complex expressions, f-strings provide a clean and readable solution.
While f-strings are powerful and convenient, it’s crucial to handle untrusted input carefully to avoid security vulnerabilities. Always validate and sanitize user input, or use safer alternatives when dealing with potentially malicious data.
If you are interested in Python development, you can find more in our Amazing Python Package Showcase
JeremyTab
static balancing machines
Static Balancing Machines: The Guardians of Rotor Harmony
In the ancient realm of mechanical engineering, where forces of nature converge with human ingenuity, static balancing machines stand as formidable guardians, ensuring that every rotor spins in perfect harmony. These machines, revered for their prowess, are essential for achieving equilibrium in a myriad of rotating components that power our world. From the blades of mighty fans to the intricate systems of turbines, static balancing machines wield the power to eradicate imbalance, thus preserving the integrity of machinery and safeguarding against untold disruptions.
The Essence of Static Balance
Static balance is akin to the stillness of a serene pond, where the center of gravity aligns perfectly with the axis of rotation. When the rotor, an embodiment of dynamic energy, resides in a state of static imbalance, it becomes a plaything of gravity. The heavy point of the rotor, like a wayward star, seeks to descend, creating a gravitational pull that threatens chaos. Thus, static balancing becomes a sacred rite, involving the meticulous addition or removal of mass at designated points on the rotor, guiding it back to its rightful celestial position.
The Dance of Dynamism
Unlike its static counterpart, the dynamic balance unfolds in a grand ballet of motion. Here, the rotor engages in a more complex performance, featuring two mass displacements in differing planes. This dynamic imbalance is a source of discordance, causing not only a one-sided force but also generating unwanted vibrations as the rotor twirls. Unlike the predictable nature of static imbalance, dynamic imbalance eludes simple remedies, demanding the intricate orchestration of forces to restore harmony. The Balanset-1A, a revered artifact in this realm, empowers operators to achieve this balance with precision, employing advanced analysis and two-plane balancing techniques.
The Journey of Balancing
The journey of dynamic balancing unfolds through a series of stages, akin to a hero’s quest. Initially, the rotor is fastened upon the pedestal of the static balancing machine, where sensors act as the vigilant sentinels, capturing the vibrations. This initial measurement serves as the foundation upon which all future calculations are built. The operator, a master of the craft, then embarks on a path of experimentation: weights are introduced, subtly altering the dynamics as the rotor spins. Vibrations ebb and flow, revealing insights into the intricate dance of forces.
Calibration: The Sacred Art
The art of calibration unfolds like a tapestry, woven with threads of knowledge and empirical data. The initial stage involves the strategic placement of a known calibration weight at a predetermined point on the rotor. As the rotor awakens, vibrations react to the new configuration, and the data gathered is meticulously logged. In the succeeding stages, the operator relocates the calibration weight, which continues to unveil the secrets of balance. This meticulous process ensures that every nuance is accounted for, paving the way for the ultimate assessment of equilibrium.
Corrective Measures: The Final Weights
Having gathered the wisdom from the first trial, the operator delves into the realm of corrective measures. This critical phase compels a deep understanding of angles and positions, akin to an ancient navigator charting a course through uncharted waters. The exact positions for corrective weights become essential knowledge, guiding the placement of compensatory masses to counteract imbalances. The final installation culminates in the sacred act of spinning the rotor once more, where the vibrations are measured anew, unveiling the fruits of labor. A victorious decrease in vibration levels signifies that harmony has been restored, a testament to the power of static balancing machines.
Tools of the Trade
In the pantheon of balancing machines, the Balanset-1A reigns supreme. This portable balancer embodies versatility, designed to tackle the challenges posed by a myriad of rotor types—crushers, fans, turbines, and beyond. Its dual-channel functionality allows for dynamic balancing in two planes, providing a comprehensive solution to industrial challenges. The Balanset-1A stands ready to serve industries seeking triumph over imbalance, executing its function with unwavering precision and reliability.
The Visual Chronicles of Balancing
A sequence of images unfolds like a mythical chronicle, capturing the essence of the rotor’s journey to balance. Each photograph encapsulates a moment—a fleeting glance into the dynamic adjustments as sensors are positioned, weights are added, and measurements are taken. The visual tales reflect the transformation of raw energy into an orchestrated dance of mechanical equilibrium, each step vital to the overarching narrative of harmony.
Beyond the Mechanical: A Philosophy of Balance
Embracing the philosophy of balance transcends beyond the mere mechanics of machinery. It symbolizes a broader essence—the harmony that exists in all aspects of existence. The pursuit of static and dynamic balance becomes a metaphor for life, where equilibrium is not only sought after in the realm of machines but also in the intricacies of our daily endeavors. Just as static balancing machines rectify physical imbalances, we too can strive for balance in our lives, cultivating a state of serenity amidst the chaos of existence.
Conclusion: The Enduring Legacy of Static Balancing Machines
In the annals of engineering, static balancing machines emerge as timeless sentinels of order within the turbulent realm of rotation. Their capacity to perceive, assess, and correct imbalances resonates with a profound understanding of mechanical phenomena. As we navigate the complexities of the industrial world, it is these machines that ensure that our rotors hum with the harmonious cadence of balance, safeguarding the integrity of machines that power our lives. The legacy of static balancing machines is etched into the fabric of engineering marvels, a testament to humanity’s relentless quest for harmony in motion.