Docker Deep Dive: A Comprehensive Guide From Installation To Publishing
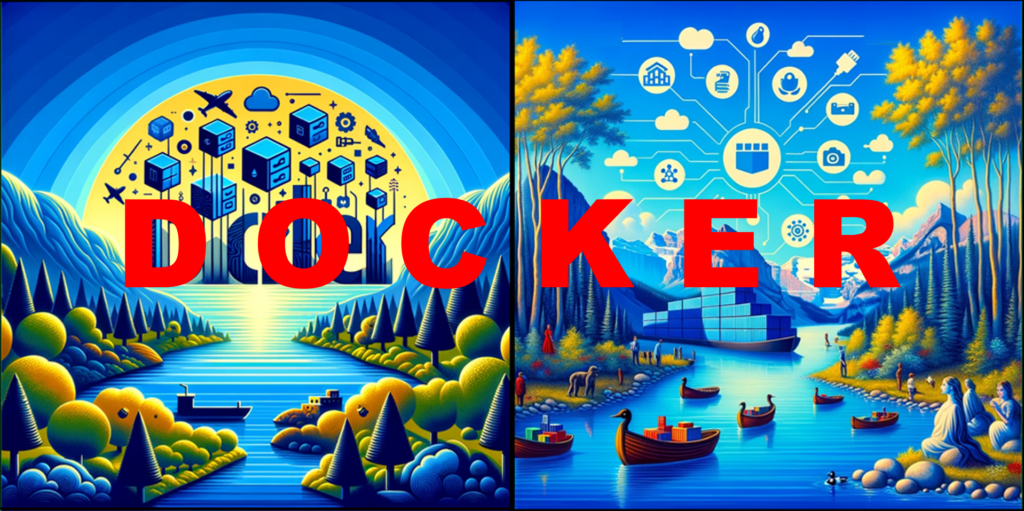
Table of Contents
Docker stands as a testament to simplicity and efficacy in the software development realm, thanks to its elegant architecture.
Quick-Start Guide: Installing Docker
Ready to dive into the Docker world? Here’s a quick guide to get you started, tailored to different operating systems:
Linux
Option 1: Using Package Manager:
Follow the official installation guide to install Docker Desktop
Option 2: Using Official Script:
- Run
curl -fsSL https://get.docker.com -o get-docker.sh
- Run
sudo sh get-docker.sh
(carefully review the script before running)
Windows
- Download and install Docker Desktop from https://www.docker.com/products/docker-desktop.
- Follow the on-screen instructions for the installation process.
macOS
Option 1: Docker Desktop:
- Download and install Docker Desktop from https://www.docker.com/products/docker-desktop.
- Follow the on-screen instructions for the installation process.
Option 2: Homebrew:
- Install Homebrew: https://brew.sh/
- Run
brew install --cask docker
% brew install --cask docker
==> Downloading https://raw.githubusercontent.com/Homebrew/homebrew-cask/8353b824e39f0293265f0cbb56de6d47450315a4/Casks/d/docker.rb
############################################### 100.0%
==> Downloading https://desktop.docker.com/mac/main/arm64/137060/Docker.dmg
############################################### 100.0%
==> Installing Cask docker
==> Moving App 'Docker.app' to '/Applications/Docker.app'
==> Linking Binary 'docker' to '/usr/local/bin/docker'
==> Linking Binary 'docker-compose' to '/usr/local/bin/docker-compose'
==> Linking Binary 'docker-credential-desktop' to '/usr/local/bin/docker-credential-desktop'
==> Linking Binary 'docker-credential-ecr-login' to '/usr/local/bin/docker-credential-ecr-login'
==> Linking Binary 'docker-credential-osxkeychain' to '/usr/local/bin/docker-credential-osxkeychain'
==> Linking Binary 'docker-index' to '/usr/local/bin/docker-index'
==> Linking Binary 'kubectl' to '/usr/local/bin/kubectl.docker'
==> Linking Binary 'docker.bash-completion' to '/opt/homebrew/etc/bash_completion.d/docker'
==> Linking Binary 'docker.zsh-completion' to '/opt/homebrew/share/zsh/site-functions/_docker'
==> Linking Binary 'docker.fish-completion' to '/opt/homebrew/share/fish/vendor_completions.d/docker.fish'
==> Linking Binary 'hub-tool' to '/usr/local/bin/hub-tool'
==> Linking Binary 'com.docker.cli' to '/usr/local/bin/com.docker.cli'
🍺 docker was successfully installed!
Verifying Installation
Once installed, run docker run hello-world
in a terminal. This should download and run a simple container, printing a confirmation message.
% docker run hello-world
Unable to find image 'hello-world:latest' locally
latest: Pulling from library/hello-world
478afc919002: Pull complete
Digest: sha256:4bd78111b6914a99dbc560e6a20eab57ff6655aea4a80c50b0c5491968cbc2e6
Status: Downloaded newer image for hello-world:latest
Hello from Docker!
This message shows that your installation appears to be working correctly.
To generate this message, Docker took the following steps:
1. The Docker client contacted the Docker daemon.
2. The Docker daemon pulled the "hello-world" image from the Docker Hub.
(arm64v8)
3. The Docker daemon created a new container from that image which runs the
executable that produces the output you are currently reading.
4. The Docker daemon streamed that output to the Docker client, which sent it
to your terminal.
To try something more ambitious, you can run an Ubuntu container with:
$ docker run -it ubuntu bash
Share images, automate workflows, and more with a free Docker ID:
https://hub.docker.com/
For more examples and ideas, visit:
https://docs.docker.com/get-started/
Note:
- Command lines might vary slightly depending on your specific Linux distribution.
- Remember to follow instructions carefully, especially when using downloaded scripts.
- Consider your needs and preferences when choosing between Docker Desktop and command-line installations.
Docker’s Fundamentals: Core Components
Docker Client
The friendly command line interface or GUI tool. It sends instructions to the Docker daemon, the workhorse behind the scenes.
Docker Daemon
The heart and soul of Docker, running in the background. It receives commands from the client, manages containers, and interacts with the underlying operating system.
Docker Objects
- Images: The blueprints containing instructions and configurations for building containers.
- Containers: The running instances of those images, isolated and self-contained environments for your applications.
- Networks: Virtual networks connecting containers, allowing them to communicate with each other.
- Volumes: Persistent storage options for containers, ensuring data survives beyond container restarts.
Docker Registry
A giant library to store and share the Docker images. Public registries like Docker Hub offer pre-built images, while private registries allow secure sharing within your organization.
Docker Engine API
The communication channel between the client and daemon. It enables other tools like Docker Compose to interact with Docker and manage complex deployments.
Orchestration Platforms (Optional)
For managing large-scale deployments, tools like Docker Swarm and Kubernetes come into play. They coordinate and scale containerized applications across multiple machines.
Harnessing Docker Hub’s Repository
Docker Hub acts as a treasure chest of pre-configured images, simplifying the setup process for numerous applications.
Search for Images
Visit https://hub.docker.com and search for the application you need. For example, search for “nginx” to find the official Nginx web server image.
Pull the Image
Once you find the desired image, use the docker pull
command to download it to your local machine. For example, to pull the Nginx image:docker pull nginx
Run the Container
You can now run the pulled image to create a container. The basic command is:docker run <image_name>
Replace <image_name>
with the actual name (e.g., docker run nginx
). This will start a new container based on the image and run the application within it.
Explore Available Images
Docker Hub offers a vast collection of images, including:
- Web servers: Apache, Nginx, PHP, Node.js
- Databases: MySQL, PostgreSQL, MongoDB
- Development tools: Git, Vim, Visual Studio Code
- Utilities: Redis, Memcached, RabbitMQ
Customize and Extend
Many images allow customization through environment variables, volumes, and command-line arguments. Refer to the image’s documentation for specific options.
Remember:
- Always check the image’s documentation for specific usage instructions and potential security considerations.
- Use trusted images from reputable sources like official maintainers or well-established organizations.
- Be mindful of resource usage, as some pre-built images can be resource-intensive.
Crafting Custom Images with Dockerfiles
Dockerfiles allow you to define the blueprint for your own custom Docker images, tailored to your specific application needs. Let’s dive into the basics of building these images.
Steps
1. Creating a Dockerfile: Create a plain text file named Dockerfile
in your project directory. This file will contain instructions for building your image layer by layer.
2. Choosing a Base Image: Start by specifying the base image for your build. This provides a starting point with an operating system and basic tools.
3. Installing Dependencies: Use the RUN
instruction to install required software packages using your system’s package manager.
4. Copying Application Code: Use the COPY
instruction to copy your application code, configuration files, and other necessary files from your host machine into the image. For example:
5. Setting Up User and Permissions: Use the USER
instruction to change the user under which the application runs and RUN
to set appropriate permissions on files and directories.
6. Defining Entry Point: Use the CMD
instruction to specify the command that will be executed when the container starts.
A Example of Dockerfile
# Use Node.js 18 slim image for smaller footprint
FROM node:18-slim
# Create working directory
WORKDIR /app
# Copy package.json and package-lock.json
COPY package*.json ./
# Install dependencies
RUN npm install
# Copy remaining application code
COPY . .
# Expose port for web services
EXPOSE 3000
# Start the application when container runs
CMD ["npm", "start"]
# Explanation:
# Base Image: Uses node:18-slim for a smaller image size.
# Working Directory: Sets /app as the working directory.
# Dependencies: Copies package.json and package-lock.json followed by running npm install to install dependencies.
# Application Code: Copies all remaining files to /app.
# Expose Port: Exposes port 3000 for web services (adjust based on your app).
# Start Command: Runs npm start to start the Express.js application.
Building the Image
Navigate to your project directory in your terminal and run the following command to build the image:
docker build -t my-custom-image .
Replace my-custom-image
with your desired image name.
Running the Image
Use the docker run
command with your image name to start a container based on the image:
docker run -p 8080:8080 my-custom-image
This will map port 8080 of the container to port 8080 on your host, making your web application accessible.
Simplifying Application Deployment with Docker Compose
Docker Compose simplifies managing intricate applications composed of multiple containers by defining them and their configurations in a single YAML file.
Docker Compose File
Docker Compose File (docker-compose.yml
) outlines all your services (containers) and their configurations. Here’s a basic example for a web application:
version: '3.8'
services:
app:
container_name: nodejs_app
build: .
ports:
- "3000:3000"
environment:
- DB_HOST=postgres
- DB_USER=postgres
- DB_PASS=mysecretpassword
- DB_NAME=mydatabase
- DB_PORT=5432
volumes:
- .:/usr/src/app
- /usr/src/app/node_modules
depends_on:
- postgres
command: npm start
postgres:
container_name: postgres_db
image: postgres:latest
ports:
- "5432:5432"
environment:
- POSTGRES_USER=postgres
- POSTGRES_PASSWORD=mysecretpassword
- POSTGRES_DB=mydatabase
volumes:
- postgres_data:/var/lib/postgresql/data
volumes:
postgres_data:
## Explanation ##
Version: Specifies the Docker Compose file format version. '3.8' is one of the latest versions and should be compatible with most features.
Services: Defines two services, app (your Node.js application) and postgres (the PostgreSQL database).
app:
container_name: Name of the container running your Node.js application.
build: Context to build the Docker image for your app. Assumes your Dockerfile is in the same directory as the docker-compose.yml.
ports: Maps port 3000 on the container to port 3000 on the host, allowing you to access the app using http://localhost:3000.
environment: Environment variables for your app, including database connection details.
volumes: Mounts the current directory into the container and keeps node_modules persistent.
depends_on: Ensures the postgres service is started before the app service.
command: Overrides the default command to run your application (e.g., npm start).
postgres:
container_name: Name of the container running PostgreSQL.
image: Specifies the PostgreSQL image version to use.
ports: Maps port 5432 on the container to port 5432 on the host.
environment: Environment variables for PostgreSQL, including the default user, password, and database.
volumes: Persists the database data using a named volume (postgres_data).
Volumes: Defines a named volume (postgres_data) to persist the database data beyond the container's lifecycle.
Key Features
- Service Definitions: Each service section details individual containers with properties like build instructions, ports, environment variables, and volumes.
- Multi-container Architecture: Manage multiple connected containers as a single application.
- Volumes: Mount host directories as persistent data volumes for containers.
- Environment Variables: Inject configuration values into containers at runtime.
- Networks: Define internal networks for container communication.
Running Docker Compose
docker-compose up
: Start all defined services in detached mode (background).docker-compose down
: Stop and remove all running services.docker-compose build
: Build images for all services defined in the file.docker-compose ps
: List running services and their status.docker-compose logs <service>
: View logs for a specific service.
Source Code Examples
The Docker Compose documentation provides numerous examples: https://docs.docker.com/compose/compose-file/.
Sharing is Caring: Publishing Docker Images
Sharing your Docker images on platforms like Docker Hub fosters collaboration and enables others to benefit from your work. Here’s how you can publish your images:
Choosing a Registry
- Docker Hub: The largest public registry, ideal for open-source projects and sharing with a broad audience. Requires a free Docker Hub account.
- Private Registries: Ideal for internal company use or paid services for increased control and security. Examples include GitLab Container Registry, AWS ECR, Azure Container Registry.
Tagging Your Image
- Use meaningful tags to identify different versions or variants of your image. Example:
my-image:latest
for the latest version.
Pushing to the Registry
- Docker Hub:
docker login
docker push your-username/my-image:latest
- Private Registries: Follow specific instructions provided by the platform (e.g., API calls, CLI tools).
Optimizing Visibility
- Write a clear and informative image description on the registry platform.
- Include usage instructions, dependencies, and links to documentation.
- Use relevant keywords and tags for discoverability.
Additional Considerations
- Security: Scan your image for vulnerabilities before publishing.
- Licensing: Specify the license terms under which your image is shared.
- Maintenance: Update your image and documentation regularly.
Leave a Reply